Near the year’s end, holidays and gatherings with family and friends have put me to postpone updating for a while. As 2022 officially ended, it’s worth spending some time to reflect on the things we have gone through and learnt. Personally, I started a new role in forex, picked up workout habits, chartered as a Financial Risk Manager, and most importantly, I decided to pivot to a more quantitative career after contemplating where my passion sits and how it matches with my personality. Since then, I have spent quite some time trying to figure out the concept of Object Orient Programming (aka OOP) when I inevitably get to the concept of Class in Python programming. Below is a summary of what I have learnt so far.
What is a class?
A class can be considered a template or blueprint of a particular user-defined data type. A class has a set of unique attributes and methods that the objects of this class can only access.
Attributes are the information uniquely meaningful to a class. For instance, a user will have a username and password to log on to his account, and it has an email address to receive and send messages. In addition, there is an account status showing whether a user is online. A user’s username, password, email address, and status are attributes of this user.
Methods are functions that uniquely belong to a class. These methods define constraints on what a class can and cannot do. The first parameter “self” in a method refers to the object calling the method.
“Dunder” (abbreviation of double-underscore) or “magic” methods are special methods that enhance the functionality of a class. These methods cannot be called explicitly. Among these dunder methods, __init__ ( ) is paramount. __init__( ) is used to initialize attributes to the object created, which is called whenever an object is instantiated from a class. It is the constructor in Python.
To illustrate, a user can register a new account, log on to his account, send messages to others, and check his current privileges on the platform. These are known as methods that the user can call to represent his behaviours.
What is an object?
An object is an instance of its class with specified data, which can be created through instantiation. The object created has access to its class methods. Taking the user example, a user called “kenneth123” is an object of the User class.
What is Object Oriented Programming?
The pattern of defining “classes” and creating “objects” to represent the responsibilities of a program is known as Object Oriented Programming. OOP is a programming paradigm, just like Functional Programming and Structured Programming. Simply put, OOP is built based on different objects. These objects contain specific data (attributes) and have access to functions (methods) that are uniquely defined. When writing a program, we can utilize the idea of OOP by creating different classes and defining their interactions.
Four important principles are inherent in OOP: Encapsulation, Abstraction, Inheritance, and Polymorphism. Let’s take a deeper look at them.
1) Encapsulation:
The detail of an object is hidden inside, and only selected information is exposed. Attributes and methods of an object are defined in its class. When an object is instantiated, these attributes and methods are encapsulated in this object. An object cannot access information from another class, and only predefined methods can be called on an object.
2) Abstraction:
Objects only reveal information relevant to the current situation, hiding unnecessary information. Attributes of an object should only be modified through its methods; this layer of abstraction enables programmers to work with the object at a high level without worrying about inner complex code.
3) Inheritance:
A class can reuse predefined code from another class. When this relationship is established, the former is called a child class or subclass of the latter one (parent class or superclass). The child class inherits attributes and methods from its parent class.
4) Polymorphism:
An object can have many forms based on its usage and share its behaviours; this principle reduces duplicated code. This is achieved by defining a child class which extends its functionality from its parent class and implements its methods differently to deal with specific tasks.
OOP comes in handy when we want to model real entities in our program, and it is clearer to understand OOP through an example.
Using the User example discussed above, we now create a User class using Python.
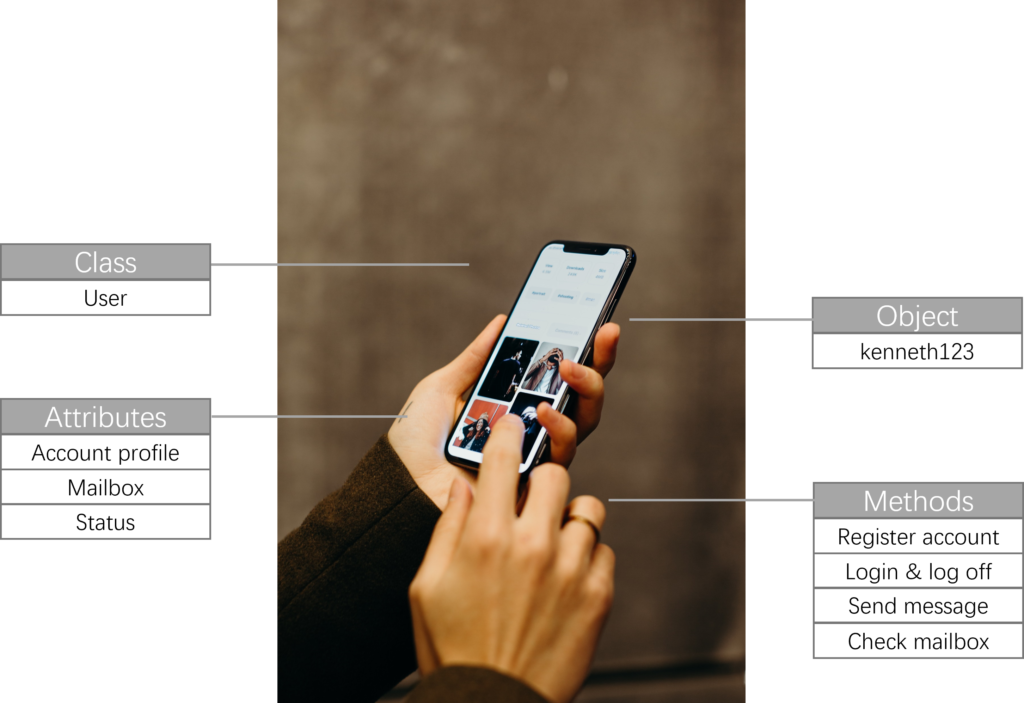
You may wonder why we did not see the “account profile” attribute when we first demonstrated the User example. As we know, a user contains username, password, and email as attributes. When our project scale grows in the future, we will find that we have a growing list of attributes of this User class. Therefore, it is sensible to break those features into a separate class.
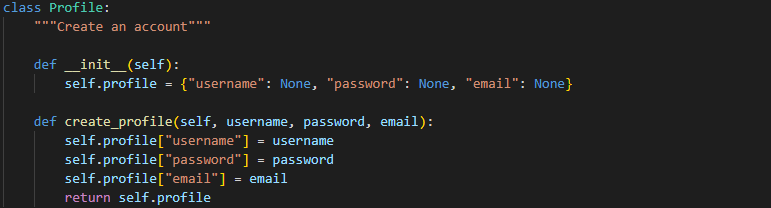
Following the same philosophy, we now create a separate class to store user’s mailbox data.

Here comes the part we define our User class. As shown below, the class has attributes named “account_profile” and “mailbox” which create instances from the Profile and Mailbox classes we defined earlier, along with a status attribute which indicates whether the user is online. The __repr__( ) method will provide a description if we try to print an object which instantiated from the User class.
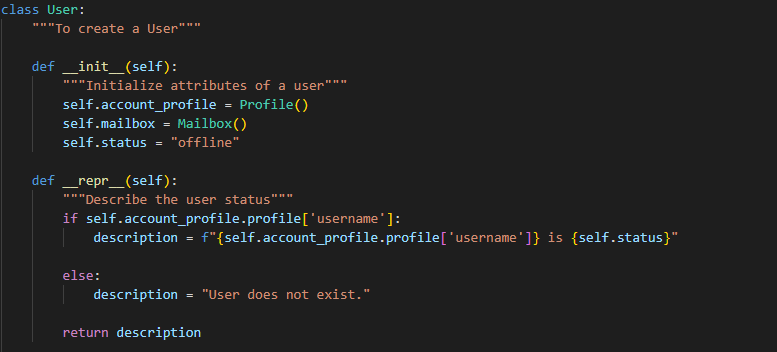
Below are the methods we created for the User class, including account registration (if the user does not exist), login, sending messages, checking the mailbox, and logging off. The program checks whether the user is logged on before calling the methods.
A platform may have multiple types of users who pay different subscription fees to enjoy different services. Let’s define three types of users, Basic, Premium, and VIP, each with different privileges. And users can check their privileges by calling the “check_privilege” method. These three types of users are created through inheritance from their common parent class “User”. The super( ) function allows the child class to access its parent class’s attributes and call methods.
The above example gives us a general idea of how OOP works in Python. It gives us the advantage of writing more robust code with reusability and security.