What is lambda function?
Lambda function is an anonymous function or function without explicit definition, which can take in multiple parameters with single line expression. Since lambda is an anonymous function not designed to be stored, assigning a lambda function to a variable is not encouraged.
lambda parameters: expression
Lambda functions are commonly used along with filter(), map(), and reduce() functions.
filter()
The filter() function extracts elements from an iterable for which a function returns True, and it returns a filter object. The filter() function is self-explanatory, and is particularly designed for filtering.
filter(function, iterable)
Example: To find the numbers greater than 20 from a given list
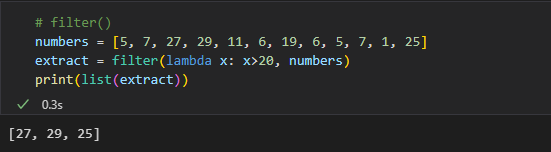
map()
The map() function transforms each elements in an iterable based on a given function and returns a map object.
map(function, iterable)
Example: To find the square of each number in a given list
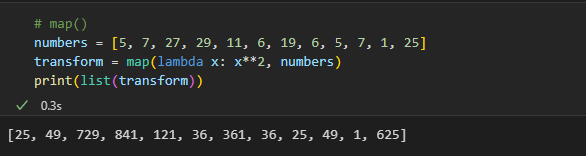
reduce()
The reduce() function applies a function of two arguments to all elements of an iterable, from left to right, to reduce the iterable cumulatively down to a single value. The return type depends on the iterable.
reduce(function, iterable)
Example: To find the product of a list of numbers
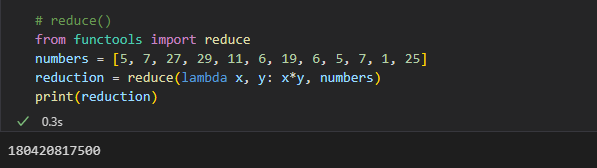
Lambda vs defined function
Defined function
While defining normal functions, we’re using the def keyword in Python. The content of the function can be as large as we want it to be and contains numerous expression. A function will perform the code we typed inside the function body, and by default, return us a None value, if we did not specify the return value.
Lambda function
It is a way to compactify the writing of our functions, which is useful when we create something that we don’t need to use repeatedly in our code. We generally use lambda function as an argument to a higher-order function. It is more frequently used in the Functional Programming paradigm.
- Lambda function is anonymous while defined function is explicit.
- Lambda function can take in one line of expression while defined function can have multiple lines of expression.
- Lambda function automatically returns the computed value while defined function requires us to specify the return value.
Example: a simple calculator
We can build a simple calculator by using the defined function and lambda function respectively.
Using defined function
Using lambda function
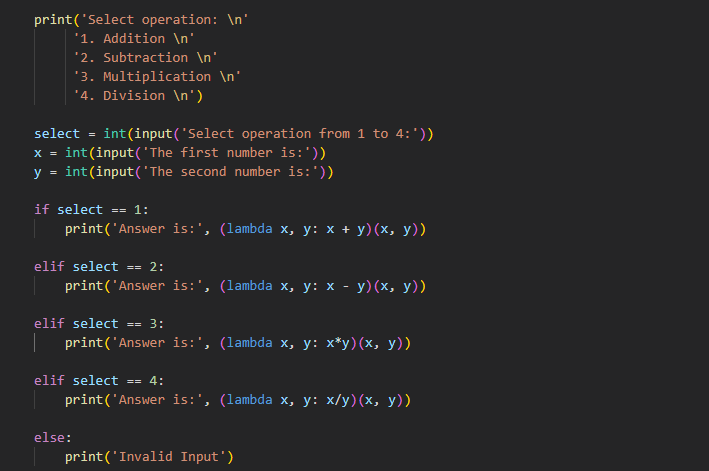